Subdomain enumeration is a critical aspect of cybersecurity, serving as the foundation for identifying potential vulnerabilities within an organization’s online infrastructure. Python and Go, both versatile programming languages, are often utilized in the development of subdomain enumeration tools. In this article, we will conduct a code-level comparison of Python and Go for subdomain enumeration, exploring the strengths and considerations of each language in this specific cybersecurity application.
Python for Subdomain Enumeration
Python‘s readability and extensive libraries make it a popular choice for developing subdomain enumeration tools. Let’s explore a basic example of subdomain enumeration using Python:
import requests
from bs4 import BeautifulSoup
def enumerate_subdomains(domain):
url = f'https://crt.sh/?q=%.{domain}&output=json'
try:
response = requests.get(url)
data = response.json()
subdomains = set()
for entry in data:
subdomains.add(entry['name_value'])
return subdomains
except Exception as e:
print(f"Error: {e}")
return set()
if __name__ == "__main__":
target_domain = "example.com"
subdomains = enumerate_subdomains(target_domain)
print(f"Subdomains for {target_domain}:")
for subdomain in subdomains:
print(subdomain)
Key Points for Python:
- Readability: Python’s clean syntax enhances code readability, making it accessible for cybersecurity professionals.
- Rich Libraries: Leveraging external libraries like
requests
for HTTP requests andBeautifulSoup
for HTML parsing streamlines the development process. - Scripting Capabilities: Python’s scripting capabilities allow for quick prototyping and automation.
Go for Subdomain Enumeration
Go’s efficiency and concurrent capabilities make it suitable for tasks like subdomain enumeration. Below is a basic example of subdomain enumeration using Go:
package main
import (
"encoding/json"
"fmt"
"io/ioutil"
"net/http"
"sync"
)
func enumerateSubdomains(domain string, wg *sync.WaitGroup) {
defer wg.Done()
url := fmt.Sprintf("https://crt.sh/?q=%%.%s&output=json", domain)
resp, err := http.Get(url)
if err != nil {
fmt.Printf("Error: %v\n", err)
return
}
defer resp.Body.Close()
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
fmt.Printf("Error reading response body: %v\n", err)
return
}
var data []map[string]interface{}
err = json.Unmarshal(body, &data)
if err != nil {
fmt.Printf("Error decoding JSON: %v\n", err)
return
}
subdomains := make(map[string]struct{})
for _, entry := range data {
subdomains[entry["name_value"].(string)] = struct{}{}
}
fmt.Printf("Subdomains for %s:\n", domain)
for subdomain := range subdomains {
fmt.Println(subdomain)
}
}
func main() {
var wg sync.WaitGroup
targetDomain := "example.com"
wg.Add(1)
go enumerateSubdomains(targetDomain, &wg)
wg.Wait()
}
Key Points for Go:
- Concurrency: Go’s native support for concurrency is evident in the use of goroutines to concurrently fetch and process subdomains, enhancing performance.
- Static Binary Compilation: Go compiles into a single binary, simplifying deployment and reducing dependencies.
- Efficiency: Go’s efficient standard library simplifies HTTP requests and JSON decoding.
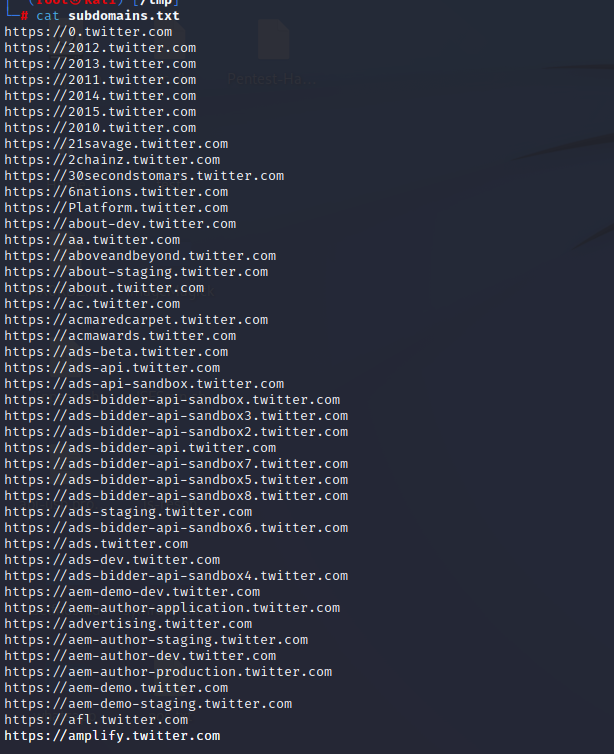
Code Comparison:
Let’s compare the key aspects of the Python and Go code for subdomain enumeration:
- Concurrency:
- Python: Lacks native support for parallelism, and concurrent execution is achieved through libraries like
asyncio
. - Go: Leverages goroutines for concurrent execution, enhancing performance in tasks like subdomain enumeration.
- Readability:
- Python: Known for its clean and readable syntax, making the code approachable for cybersecurity professionals.
- Go: Follows a minimalist design philosophy, resulting in clear and concise code, although the syntax may be less familiar to some.
- Performance:
- Python: Interpreted nature may result in slower performance, especially in CPU-bound tasks.
- Go: Compiled nature and efficient concurrency make it performant, particularly in tasks demanding parallelism.
- Dependencies:
- Python: Requires external libraries like
requests
andBeautifulSoup
. - Go: Produces a standalone binary, minimizing external dependencies and simplifying deployment.
- Error Handling:
- Python: Utilizes exceptions for error handling.
- Go: Emphasizes explicit error handling through return values.
Conclusion:
Both Python and Go offer viable solutions for subdomain enumeration in cybersecurity. Python excels in readability, extensive libraries, and scripting capabilities, making it suitable for quick development and prototyping. On the other hand, Go’s native concurrency, static binary compilation, and efficiency position it as a strong contender for performance-intensive tasks.
The choice between Python and Go ultimately depends on the specific requirements of the cybersecurity task, the preferences of the development team, and the desired trade-offs between readability and performance. Whether opting for the readability of Python or the efficiency of Go, cybersecurity professionals have powerful tools at their disposal for effective subdomain enumeration.
Leave a Reply