RSA (Rivest-Shamir-Adleman) encryption, as a cornerstone of modern cryptography, often becomes a focal point during rsa penetration testing. Ethical hackers, also known as penetration testers, simulate cyberattacks to identify vulnerabilities and weaknesses in systems. RSA’s prominence in securing critical systems and communication channels makes it a key target for assessment in penetration testing. Let’s explore where RSA might be encountered and assessed during a penetration test.
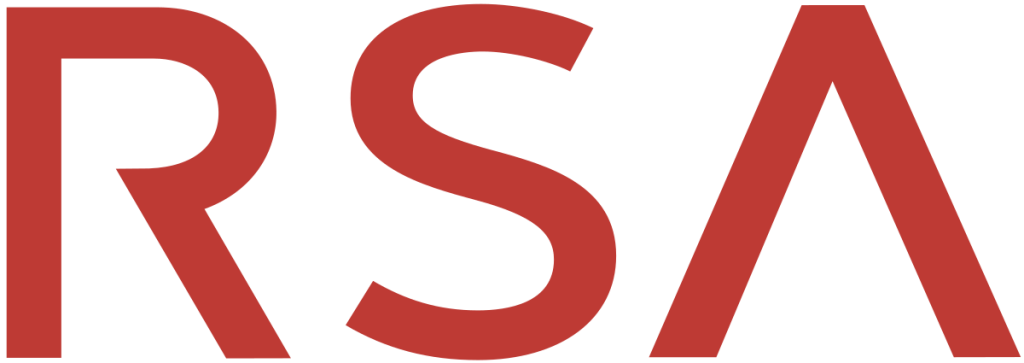
1. Key Length Analysis – Rsa Penetration Testing
One of the primary areas where RSA comes under scrutiny is the analysis of key lengths. Penetration testers evaluate the strength of RSA implementations by examining the size of the key pairs used for encryption and decryption. Shorter key lengths may be susceptible to attacks like brute force or factorization. The assessment aims to ensure that the key lengths meet current security standards, considering advancements in computational power.
Code :
# Python code to check RSA key length
from Crypto.PublicKey import RSA
key = RSA.generate(2048) # Generate a 2048-bit RSA key pair
print(f"RSA Key Length: {key.size_in_bits()} bits")
2. Cryptanalysis – Rsa Penetration Testing
Penetration testers may delve into cryptanalysis to evaluate the cryptographic protocols employing RSA. This involves examining padding schemes, random number generation, and other factors that could impact the security of the encryption. Understanding the intricacies of RSA implementation is crucial for identifying potential vulnerabilities and ensuring robust protection against unauthorized access.
Code :
# Python code for RSA cryptanalysis assessment
# (Example: Analyzing RSA padding scheme)
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
# Load public key
public_key = RSA.import_key(open('public.pem').read())
# Encrypt a message using RSA
cipher = PKCS1_OAEP.new(public_key)
ciphertext = cipher.encrypt(b'Sensitive message')
# Penetration tester analyzes padding scheme
# ...
3. Side-Channel Attacks – Rsa Penetration Testing
Sophisticated penetration tests may involve side-channel attacks on RSA implementations. Side-channel attacks exploit information leaked during the cryptographic process, such as timing information or power consumption, to gain insights into the private key. Assessing the system’s resistance to side-channel attacks is crucial for ensuring the overall security of RSA-protected systems.
Code
# Python code for side-channel attack assessment
# (Example: Timing attack on RSA)
import time
def perform_timing_attack():
start_time = time.time()
# ... RSA operations ...
end_time = time.time()
elapsed_time = end_time - start_time
return elapsed_time
# Penetration tester analyzes the timing of RSA operations
# ...
4. Implementation Flaws – Rsa Penetration Testing
Assessing the security of RSA implementations includes scrutinizing the code for implementation flaws. Penetration testers review the source code, ensuring that best practices are followed and there are no vulnerabilities that could be exploited. Common implementation flaws may include insecure key storage, improper use of random number generators, or mismanagement of cryptographic operations.
Code :
# Python code for RSA implementation flaws assessment
# (Example: Checking for secure key storage)
import os
def store_private_key_insecurely(key):
# Insecure key storage example (for illustration purposes)
with open('insecure_private_key.pem', 'w') as file:
file.write(key.export_key())
# Penetration tester identifies insecure key storage practices
# ...
Conclusion:
RSA encryption presents both challenges and opportunities for ethical hackers. Assessing RSA implementations requires a combination of cryptographic expertise, coding analysis, and a thorough understanding of the specific context in which RSA is employed. By scrutinizing key lengths, cryptanalyzing protocols, evaluating resistance to side-channel attacks, and identifying implementation flaws, penetration testers play a crucial role in enhancing the overall security posture of systems relying on RSA encryption. As technology continues to evolve, the role of penetration testers in uncovering and mitigating potential vulnerabilities in RSA implementations remains paramount for ensuring the robustness of digital security.
Leave a Reply