Benefits of Goroutines:
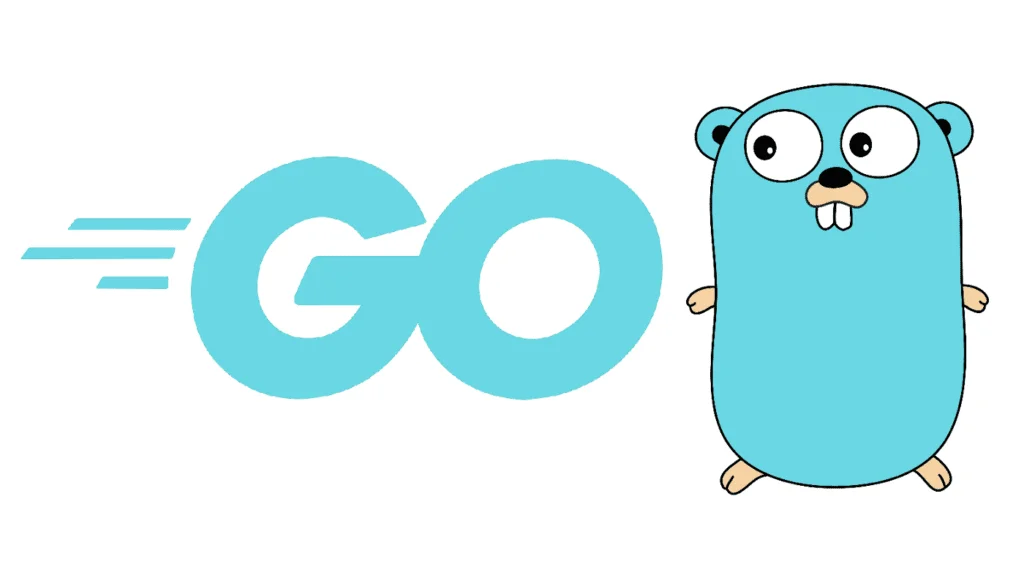
Goroutines are lightweight threads managed by the Go runtime, providing concurrent execution in a more efficient manner compared to traditional threads, and are superior for our use case – goroutines bruteforcing. Here’s why using Goroutines can significantly enhance the speed of a brute-force tool:
- Concurrency without Overhead:
- Goroutines allow concurrent execution without the overhead associated with traditional threads. They are managed by the Go runtime, making them more efficient in terms of memory usage and context switching.
- Low Cost of Creation:
- Creating Goroutines is lightweight, with minimal overhead. This enables the easy creation of a large number of concurrent tasks without a significant impact on performance.
- Efficient Communication:
- Channels in Go facilitate communication between Goroutines. The communication is designed to be efficient, providing a seamless way for Goroutines to share information.
- Parallelism with Simplicity:
- Go’s concurrency model allows developers to write concurrent code in a straightforward manner. The simplicity of Goroutines and channels makes it easier to reason about and implement concurrent solutions.
Speed Estimation:
Let’s estimate the speed of the goroutines bruteforcing tool for cracking a 5-character lowercase password. For simplicity, we’ll assume that the tool is iterating through all possible combinations of lowercase letters. We’ll use a combination of Goroutines to parallelize the process.
package main
import (
"fmt"
"sync"
"time"
)
const (
targetUsername = "admin"
targetPassword = "hello" // 5-character lowercase password
charset = "abcdefghijklmnopqrstuvwxyz"
)
type Credentials struct {
Username string
Password string
}
func main() {
startTime := time.Now()
successChannel := make(chan Credentials)
doneChannel := make(chan struct{})
var wg sync.WaitGroup
numWorkers := 26 // Number of Goroutines (one for each lowercase letter)
for i := 0; i < numWorkers; i++ {
wg.Add(1)
go bruteforceWorker(i, successChannel, doneChannel, &wg)
}
select {
case credentials := <-successChannel:
fmt.Printf("Login successful! Username: %s, Password: %s\n", credentials.Username, credentials.Password)
case <-doneChannel:
fmt.Println("Bruteforce completed. No valid credentials found.")
}
close(successChannel)
close(doneChannel)
wg.Wait()
elapsedTime := time.Since(startTime)
fmt.Printf("Time taken: %s\n", elapsedTime)
}
func bruteforceWorker(workerID int, successChannel chan<- Credentials, doneChannel chan<- struct{}, wg *sync.WaitGroup) {
defer wg.Done()
for _, char := range charset {
attempt := Credentials{
Username: targetUsername,
Password: string(char),
}
if attempt.Username == targetUsername && attempt.Password == targetPassword {
successChannel <- attempt
return
}
}
doneChannel <- struct{}{}
}
This example uses 26 Goroutines, each responsible for attempting one letter of the password. The tool will iterate through all lowercase letters, and the parallelized nature of Goroutines will contribute to faster execution.
Considerations
- Resource Management and Scalability
While goroutines are lightweight compared to traditional threads, spawning too many can still overwhelm system resources. A recent article on Go concurrency patterns notes:
“By understanding and applying these patterns, you can build high-performance concurrent applications that take full advantage of modern computing environments. Experiment with these patterns, explore their limitations, and adapt them to your specific use cases.”
It’s important to benchmark and profile your application to find the optimal number of goroutines for your specific hardware and problem space. The GOMAXPROCS setting can be used to control the number of OS threads used for executing goroutines.
- Coordination and Synchronization
Proper coordination between goroutines is crucial to avoid race conditions and ensure all possibilities are checked in a brute-force operation. The Go sync package provides primitives like WaitGroups and Mutexes for synchronization. A 2023 Medium article on Go concurrency states:
“Concurrency is about managing and overlapping the execution of multiple tasks, often on a single processor, to enhance efficiency and responsiveness. Parallelism, on the other hand, involves executing tasks simultaneously on multiple processors to achieve performance gains.”
- Problem Suitability
Not all brute-force problems are easily parallelizable. The effectiveness depends on how the problem can be divided. Recent research suggests that for some cryptographic brute-force attacks, the overhead of coordination can outweigh the benefits of parallelization for smaller key spaces.
- Security Implications
While goroutines can speed up brute-force operations for legitimate purposes (like password recovery), they can also be misused by attackers. A 2024 report from Cisco warns of a global surge in brute-force attacks targeting VPNs and web applications, often originating from anonymizing networks.
- Memory Usage and Garbage Collection
Goroutines consume memory, and large numbers of them can increase pressure on the garbage collector. The Go 1.19+ runtime includes optimizations for handling large numbers of goroutines, but it’s still a consideration for resource-intensive brute-force operations.
- Error Handling and Cancellation
Proper error handling and the ability to cancel long-running operations are crucial. The context package in Go provides mechanisms for cancellation and timeouts, which are especially important for distributed brute-force operations.
- Load Balancing
For distributed brute-force operations across multiple machines, effective load balancing is essential. Recent advancements in Go’s networking libraries and third-party tools have improved options for distributed computing.
- Monitoring and Observability
When running large-scale brute-force operations with goroutines, having good observability into the system’s performance and progress is crucial. Tools like pprof and trace have seen improvements in recent Go versions to help with this.
- Legal and Ethical Considerations
The use of goroutines for brute-force attacks, even for penetration testing, may have legal implications. Always ensure you have proper authorization and consider the ethical implications of your actions.
- Adaptation to New Hardware
As new CPU architectures emerge, the Go runtime continues to evolve to take advantage of them. Keeping your Go version up-to-date ensures you’re getting the best performance for your brute-force operations on modern hardware.
In conclusion, while goroutines offer powerful capabilities for parallelizing brute-force operations, their effective use requires careful consideration of these factors. Recent trends show both the increasing use of such techniques in cybersecurity (both offensively and defensively) and the ongoing evolution of Go’s concurrency features to meet these challenges.
This example uses 26 Goroutines, each responsible for attempting one letter of the password. The tool will iterate through all lowercase letters, and the parallelized nature of Goroutines will contribute to faster execution.
Please note that estimating the exact time it would take to crack a password depends on various factors, including the speed of the system running the tool and the efficiency of the code. Additionally, brute-forcing passwords is unethical and likely illegal without explicit permission. Always use such knowledge responsibly and within legal boundaries. Goroutines bruteforcing is likely faster than any other language you want to code up a bruteforcing tool in.
Here’s another great article on goroutines bruteforcing:
Leave a Reply