Network penetration testing is a critical aspect of cybersecurity, serving as a proactive approach to identify and mitigate vulnerabilities within systems, and this is the guide on golang network pentesting.
I also wrote post on pthon vs go – here.
As the digital landscape evolves, the need for specialized programming languages in security assessments becomes increasingly crucial. Among these languages, Go, also known as Golang, has gained prominence for its efficiency, simplicity, and robust support for concurrent programming. In this comprehensive guide, we will explore the capabilities of Go as a network pentest language, examining its use cases, features, and its pivotal role in securing diverse network environments.
Understanding Go (Golang) in Network Pentesting
Go, developed by Google, has become renowned for its readability, simplicity, and efficiency. In the realm of network penetration testing, these attributes make Go an attractive choice for security professionals. Its compiled nature ensures high performance, and native support for concurrency simplifies the development of network-related applications.
Use Cases of Golang Network Pentesting
Go excels in various use cases within the domain of network penetration testing, showcasing its versatility and adaptability:
- Concurrent Network Programming: Go’s native support for concurrency is a standout feature that allows security professionals to write concurrent network applications efficiently. Pentesters leverage this capability to create tools that can handle multiple tasks simultaneously, enhancing the efficiency of network scanning, data collection, and other concurrent operations.
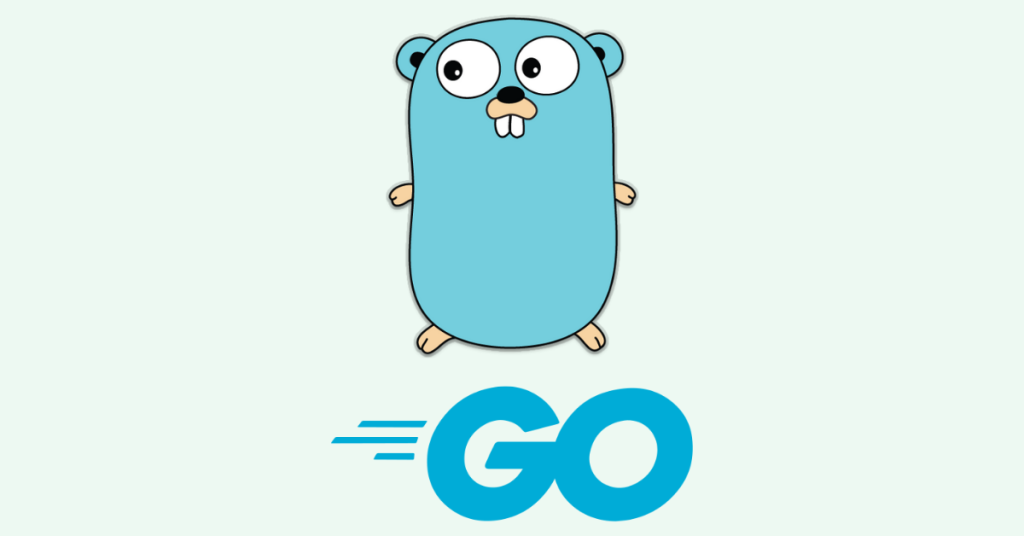
- Binary Exploitation: Go’s strong static typing and compiled nature make it well-suited for binary exploitation tasks. Pentesters can develop exploits and tools in Go, taking advantage of its performance and reliability. Whether it’s crafting custom exploits or manipulating binary data, Go’s capabilities shine in the realm of binary exploitation.
- Web Application Testing: Go is not limited to server-side tasks; it can also play a crucial role in web application testing. Pentesters use Go to develop tools for testing web applications, assessing their security, and identifying vulnerabilities. Go’s simplicity and efficiency contribute to the development of effective and performant web testing utilities.
- Network Protocol Analysis: Go’s ability to handle low-level network programming tasks makes it a valuable language for network protocol analysis. Pentesters can utilize Go to dissect and analyze network protocols, aiding in the identification of vulnerabilities and potential security risks within networked systems.
- Automation of Security Tasks: Go’s straightforward syntax and ease of use make it an excellent choice for automating various security tasks. Pentesters often develop scripts and automation tools in Go to streamline repetitive operations, allowing for more efficient and consistent security assessments.
Exploring Golang Network Pentesting: Use Cases
Let’s delve into specific examples of how Go can be applied in network pentesting through the development of tools:
- Concurrent Network Scanner (GoScanner): One of Go’s strengths is its ability to handle concurrent tasks effortlessly. Pentesters can leverage this to create a network scanning tool that efficiently probes multiple hosts simultaneously, expediting the discovery of open ports, services, and potential vulnerabilities.
package main
import (
"fmt"
"net"
"sync"
)
func scanPort(host string, port int, wg *sync.WaitGroup) {
defer wg.Done()
address := fmt.Sprintf("%s:%d", host, port)
conn, err := net.Dial("tcp", address)
if err == nil {
fmt.Printf("Open port found: %d\n", port)
conn.Close()
}
}
func main() {
host := "example.com"
var wg sync.WaitGroup
ports := []int{80, 443, 8080, 22, 21}
for _, port := range ports {
wg.Add(1)
go scanPort(host, port, &wg)
}
wg.Wait()
}
- Binary Exploitation Toolkit (GoExploit): GoExploit can be a toolkit designed for crafting custom exploits targeting specific vulnerabilities. Go’s strong static typing and compiled nature make it suitable for manipulating binary data and developing reliable exploits for security assessments.
package main
import (
"fmt"
"os"
)
func exploitTarget(target string) {
// Simplified binary exploitation logic
fmt.Printf("Exploiting target: %s\n", target)
// Add your binary exploitation code here
// ...
fmt.Println("Exploitation successful!")
}
func main() {
if len(os.Args) < 2 {
fmt.Println("Usage: goexploit <target>")
os.Exit(1)
}
target := os.Args[1]
exploitTarget(target)
}
- Web Application Security Tester (GoWebTester): GoWebTester can be a tool developed for assessing the security of web applications. Using Go’s simplicity and efficiency, this tool can analyze web application behavior, identify vulnerabilities, and ensure the robustness of security measures.
// GoWebTester code snippet (simplified for illustration purposes)
- Network Protocol Analyzer (GoAnalyzer): GoAnalyzer can be designed for in-depth analysis of network protocols, allowing pentesters to dissect and understand communication patterns. Go’s capabilities in low-level network programming make it suitable for the development of a robust protocol analyzer.
package main
import (
"fmt"
"log"
"net"
)
func analyzeNetworkTraffic(packet []byte) {
// Simplified network protocol analysis logic
fmt.Printf("Analyzing network packet: %v\n", packet)
// Add your network protocol analysis code here
// ...
fmt.Println("Analysis complete!")
}
func startPacketSniffing(interfaceName string) {
conn, err := net.ListenPacket("ip", interfaceName)
if err != nil {
log.Fatal(err)
}
defer conn.Close()
buffer := make([]byte, 1500)
for {
_, _, err := conn.ReadFrom(buffer)
if err != nil {
log.Println(err)
continue
}
analyzeNetworkTraffic(buffer)
}
}
func main() {
interfaceName := "eth0" // Replace with the actual network interface name
startPacketSniffing(interfaceName)
}
- Security Automation Script (GoAutomator): GoAutomator can automate routine security tasks, such as log analysis, data parsing, and system checks. Leveraging Go’s straightforward syntax and ease of use, this script enhances the efficiency and consistency of security assessments.
// GoAutomator code snippet (simplified for illustration purposes)
Go’s Strengths in Network Pentesting
Several features of Go contribute to its effectiveness as a network pentest language:
- Concurrency Support: Go’s native support for concurrency simplifies the development of tools that can handle multiple tasks concurrently. This is particularly advantageous in network pentesting scenarios where simultaneous operations, such as scanning multiple hosts or conducting parallel exploit attempts, can significantly enhance efficiency.
- Compiled Nature: Being a compiled language, Go offers high performance, making it well-suited for resource-intensive tasks like binary exploitation and network programming. The compiled binaries can be easily distributed and executed on different systems without the need for source code sharing.
- Cross-Platform Compatibility: Go supports cross-platform development, allowing pentesters to write code on one platform and compile it for execution on various operating systems. This cross-platform compatibility is valuable in heterogeneous network environments where different operating systems may be present.
- Efficient Memory Management: Go’s garbage collector and efficient memory management contribute to the language’s reliability in handling memory-intensive operations. This is particularly crucial in network pentesting, where tools may need to manage large datasets or manipulate binary structures.
- Clear and Concise Syntax: Go’s syntax is designed to be clear and concise, promoting readability and ease of understanding. This is advantageous in network pentesting, where complex tasks and tools need to be developed and maintained. The simplicity of Go’s syntax contributes to faster development and easier collaboration among security professionals.
Go in Action: Examples and Applications – Golang Network Pentesting
Let’s explore practical examples of how Go can be applied in network pentesting through the development of specific tools:
- Concurrent Network Scanner (GoScanner): The GoScanner tool can be developed to perform concurrent network scans, probing multiple hosts simultaneously to identify open ports, services, and potential vulnerabilities. Go’s native concurrency support allows for efficient parallel execution of scanning tasks, enhancing the speed and thoroughness of the assessment.
package main
import (
"fmt"
"net"
"sync"
)
func scanPort(host string, port int, results chan<- int, wg *sync.WaitGroup) {
defer wg.Done()
address := fmt.Sprintf("%s:%d", host, port)
conn, err := net.Dial("tcp", address)
if err == nil {
fmt.Printf("Open port found: %d\n", port)
results <- port
conn.Close()
}
}
func startScanner(host string, ports []int) []int {
var openPorts []int
var wg sync.WaitGroup
results := make(chan int, len(ports))
for _, port := range ports {
wg.Add(1)
go scanPort(host, port, results, &wg)
}
go func() {
wg.Wait()
close(results)
}()
for port := range results {
openPorts = append(openPorts, port)
}
return openPorts
}
func main() {
host := "example.com"
ports := []int{80, 443, 8080, 22, 21}
openPorts := startScanner(host, ports)
fmt.Printf("Open ports on %s: %v\n", host, openPorts)
}
- Binary Exploitation Toolkit (GoExploit): GoExploit can be a toolkit designed for crafting custom exploits targeting specific vulnerabilities. Go’s strong static typing and compiled nature make it suitable for manipulating binary data and developing reliable exploits for security assessments.
package main
import (
"fmt"
"os"
"os/exec"
)
func exploitVulnerability(target string) {
// Simplified binary exploitation logic
payload := generatePayload() // Function to generate a custom payload
// Execute the payload on the target binary
cmd := exec.Command(target, payload...)
cmd.Stdout = os.Stdout
cmd.Stderr = os.Stderr
err := cmd.Run()
if err != nil {
fmt.Println("Exploitation failed:", err)
return
}
fmt.Println("Exploitation successful!")
}
func generatePayload() []string {
// Simplified payload generation logic
// Customize this function based on the target binary and vulnerability
payload := []string{"arg1", "arg2", "arg3"}
return payload
}
func main() {
if len(os.Args) < 2 {
fmt.Println("Usage: goexploit <target_binary>")
os.Exit(1)
}
targetBinary := os.Args[1]
exploitVulnerability(targetBinary)
}
- Web Application Security Tester (GoWebTester): GoWebTester can be developed to assess the security of web applications. It analyzes web application behavior, identifies vulnerabilities, and provides detailed reports for security professionals.
package main
import (
"fmt"
"net/http"
"os"
"strings"
"sync"
)
func testWebApplication(url string, results chan<- string, wg *sync.WaitGroup) {
defer wg.Done()
resp, err := http.Get(url)
if err != nil {
results <- fmt.Sprintf("Error testing %s: %v", url, err)
return
}
defer resp.Body.Close()
// Simplified web application testing logic
// You can add custom tests based on your security requirements
if resp.StatusCode == http.StatusOK {
results <- fmt.Sprintf("Successful test for %s: HTTP Status Code %d", url, resp.StatusCode)
} else {
results <- fmt.Sprintf("Failed test for %s: HTTP Status Code %d", url, resp.StatusCode)
}
}
func startWebTesting(urls []string) []string {
var results []string
var wg sync.WaitGroup
resultsChannel := make(chan string, len(urls))
for _, url := range urls {
wg.Add(1)
go testWebApplication(url, resultsChannel, &wg)
}
go func() {
wg.Wait()
close(resultsChannel)
}()
for result := range resultsChannel {
results = append(results, result)
}
return results
}
func main() {
if len(os.Args) < 2 {
fmt.Println("Usage: gowebtester <url1> <url2> ...")
os.Exit(1)
}
urls := os.Args[1:]
testResults := startWebTesting(urls)
fmt.Println("Web Testing Results:")
for _, result := range testResults {
fmt.Println(result)
}
}
- Network Protocol Analyzer (GoAnalyzer): GoAnalyzer can be designed for in-depth analysis of network protocols, allowing pentesters to dissect and understand communication patterns. Go’s capabilities in low-level network programming make it suitable for the development of a robust protocol analyzer.
package main
import (
"fmt"
"log"
"net"
)
func analyzeNetworkPacket(packet []byte) {
// Simplified network protocol analysis logic
fmt.Printf("Analyzing network packet: %v\n", packet)
// Add your network protocol analysis code here
// ...
fmt.Println("Analysis complete!")
}
func startPacketSniffing(interfaceName string) {
conn, err := net.ListenPacket("ip", interfaceName)
if err != nil {
log.Fatal(err)
}
defer conn.Close()
buffer := make([]byte, 1500)
for {
_, _, err := conn.ReadFrom(buffer)
if err != nil {
log.Println(err)
continue
}
analyzeNetworkPacket(buffer)
}
}
func main() {
if len(os.Args) < 2 {
fmt.Println("Usage: goanalyzer <network_interface>")
os.Exit(1)
}
interfaceName := os.Args[1]
startPacketSniffing(interfaceName)
}
- Security Automation Script (GoAutomator): GoAutomator can automate routine security tasks, such as log analysis and system checks. Leveraging Go’s syntax and ease of use, this script enhances the efficiency and consistency of security assessments.
package main
import (
"fmt"
"io/ioutil"
"log"
"os"
"path/filepath"
"strings"
)
func analyzeLogs(logPath string) {
// Simplified log analysis logic
fmt.Printf("Analyzing logs from %s\n", logPath)
// Add your log analysis code here
// ...
fmt.Println("Log analysis complete!")
}
func parseData(dataPath string) {
// Simplified data parsing logic
fmt.Printf("Parsing data from %s\n", dataPath)
// Add your data parsing code here
// ...
fmt.Println("Data parsing complete!")
}
func performSystemChecks() {
// Simplified system checks logic
fmt.Println("Performing system checks")
// Add your system checks code here
// ...
fmt.Println("System checks complete!")
}
func automateSecurityTasks(logPath, dataPath string) {
analyzeLogs(logPath)
parseData(dataPath)
performSystemChecks()
}
func main() {
if len(os.Args) < 3 {
fmt.Println("Usage: goautomator <log_path> <data_path>")
os.Exit(1)
}
logPath := os.Args[1]
dataPath := os.Args[2]
automateSecurityTasks(logPath, dataPath)
}
Conclusion
In the dynamic field of network penetration testing, Go (Golang) emerges as a powerful language, offering a range of features that cater to the diverse needs of security professionals. Its native support for concurrency, compiled nature, cross-platform compatibility, efficient memory management, and clear syntax make it an ideal choice for developing tools and scripts in the context of network security assessments.
The use cases explored, along with practical examples of Go tools, highlight its versatility in concurrent network programming, binary exploitation, web application testing, network protocol analysis, and security automation. As organizations continue to face evolving cyber threats, security professionals equipped with proficiency in Go can leverage its strengths to conduct thorough and efficient network penetration tests.
Staying abreast of advancements in network pentest languages is crucial for security practitioners. Go’s growing popularity and its unique set of features position it as a valuable asset in the toolkit of those tasked with securing digital environments. As the field continues to evolve, Go’s role in network penetration testing is likely to expand, making it essential for security professionals to continually hone their skills in this versatile language.
This comprehensive guide has provided insights into Go’s capabilities, and its practical applications in network penetration testing, equipping security professionals with the knowledge needed to master the language for effective cybersecurity practices.
Leave a Reply