The ubiquitous Basic Authentication prompt is a familiar sight on web applications, offering a lightweight authentication scheme with a simple username and password mechanism. In this extensive exploration, we will delve into the nuances of Basic Authentication, shedding light on its mechanisms, vulnerabilities, and best practices.
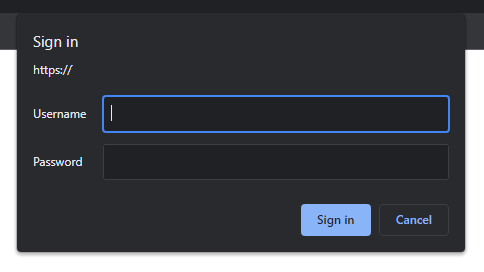
Understanding Basic Authentication:
Basic Authentication serves as a minimalistic security measure, allowing administrators to fortify web applications through a straightforward username and password mechanism. While suitable for non-critical applications, it falls short of meeting contemporary standards for secure applications, often being considered inferior and obsolete when compared to more advanced session management schemes like cookie-based authentication.
Basic Auth Example:
Upon successful authentication, a web browser appends a specific header to each subsequent request, exemplified by the following snippet:
Authorization: Basic dXNlcm5hbWU6cGFzc3dvcmQ=
This Base64-encoded string, dXNlcm5hbWU6cGFzc3dvcmQ=
, decodes to the familiar format of username:password
.
Realms in Basic Authentication:
Basic Authentication introduces the concept of ‘realms,’ allowing administrators to segregate different areas of an application, each protected by distinct credentials. This feature facilitates the use of varied databases and credential sets for different parts of the application. However, for most configurations, realms are optional and may not be necessary.
Security Implications of Basic Authentication:
While Basic Authentication can be implemented securely, inherent behaviors often raise concerns. Some notable security risks associated with Basic Authentication include:
- Transmission of credentials in every request: Despite Base64 encoding, the ease of decoding poses a security risk.
- Lack of password brute force protection: Many Basic Authentication configurations lack safeguards against password brute forcing, potentially exposing external-facing systems to prolonged attacks.
- Absence of logout functionality: Logout support is not universal, necessitating workarounds that may not be compatible with all browsers.
- Limited password reset capabilities: Basic Authentication implementations often lack user-friendly mechanisms for password resets, creating challenges for users who need immediate assistance.
Pentesting Basic Authentication:
Pentesting Basic Authentication is a critical step in securing web applications. This process involves actively testing the security of Basic Authentication implementations to identify and mitigate potential vulnerabilities. Let’s explore various techniques and tools to perform effective pentesting on Basic Authentication.
Leveraging Tools for Pentesting Basic Authentication:
Pentesters can enhance their evaluation by leveraging tools like Burp Suite, a powerful platform for web application security testing. Within Burp Suite’s User Options, credentials can be stored to streamline the testing process and prevent repetitive logouts. This approach significantly improves efficiency during pentesting Basic Authentication scenarios.
Pentesting Basic Authentication with Burp Suite:
1. [Link to Burp Suite](#)
2. Configure User Options to store credentials for seamless testing.
For situations necessitating Basic Auth credentials with curl
, the following command can be utilized:
curl -u username:password http://
# Python script to demonstrate Basic Auth headers
import requests
url = "http://example.com"
headers = {'Authorization': 'Basic dXNlcm5hbWU6cGFzc3dvcmQ='}
response = requests.get(url, headers=headers)
print(response.text)
Pentesting Basic Authentication with Python:
Pentesters often resort to scripting languages like Python to automate and customize pentesting Basic Authentication scenarios. The following Python script demonstrates the process:
# Python script for Basic Authentication pentesting
import requests
def basic_auth_pentest(username, password):
url = "http://example.com"
headers = {'Authorization': f'Basic {base64.b64encode(f"{username}:{password}".encode()).decode()}'}
response = requests.get(url, headers=headers)
print(response.text)
# Usage
basic_auth_pentest("username", "password")
This Python script sends a request with Basic Authentication headers, allowing pentesters to test various credentials programmatically.
Pentesting Basic Authentication with Go:
For a compiled language like Go, a similar approach can be employed. The following Go code snippet illustrates pentesting Basic Authentication using the popular net/http
package:
package main
import (
"encoding/base64"
"fmt"
"net/http"
)
func main() {
username := "username"
password := "password"
url := "http://example.com"
authHeader := "Basic " + base64.StdEncoding.EncodeToString([]byte(username+":"+password))
req, err := http.NewRequest("GET", url, nil)
if err != nil {
fmt.Println("Error creating request:", err)
return
}
req.Header.Add("Authorization", authHeader)
client := http.Client{}
resp, err := client.Do(req)
if err != nil {
fmt.Println("Error making request:", err)
return
}
defer resp.Body.Close()
// Handle response
fmt.Println("Response:", resp.Status)
}
This Go code performs pentesting Basic Authentication using an HTTP GET request with the necessary headers.
In conclusion, Basic Authentication, while prevalent, requires careful consideration and evaluation, especially in the context of security testing. By understanding its mechanisms, vulnerabilities, and best practices, administrators and pentesters can navigate the nuances of Basic Authentication to ensure a robust and secure web application environment.
This expanded exploration has provided a detailed insight into Basic Authentication, emphasizing its strengths and weaknesses, and offering practical tips for effective pentesting Basic Authentication. As the digital landscape evolves, staying informed about authentication mechanisms remains crucial for maintaining a secure online environment.
Leave a Reply